API Examples
Info.
This page will continue to be updated!
How to use Dart API
Dart-API is a package API included in Dart-SDK. Dart-API contains many APIs, and each API has a different function and a different code line. When users code an app, they will call API which fits their purpose.
This is an example of how to call the API 'Get the name of connected robot model.'
As you can see in the dart_api.ts
file, it provides getRobotModel
API as below.
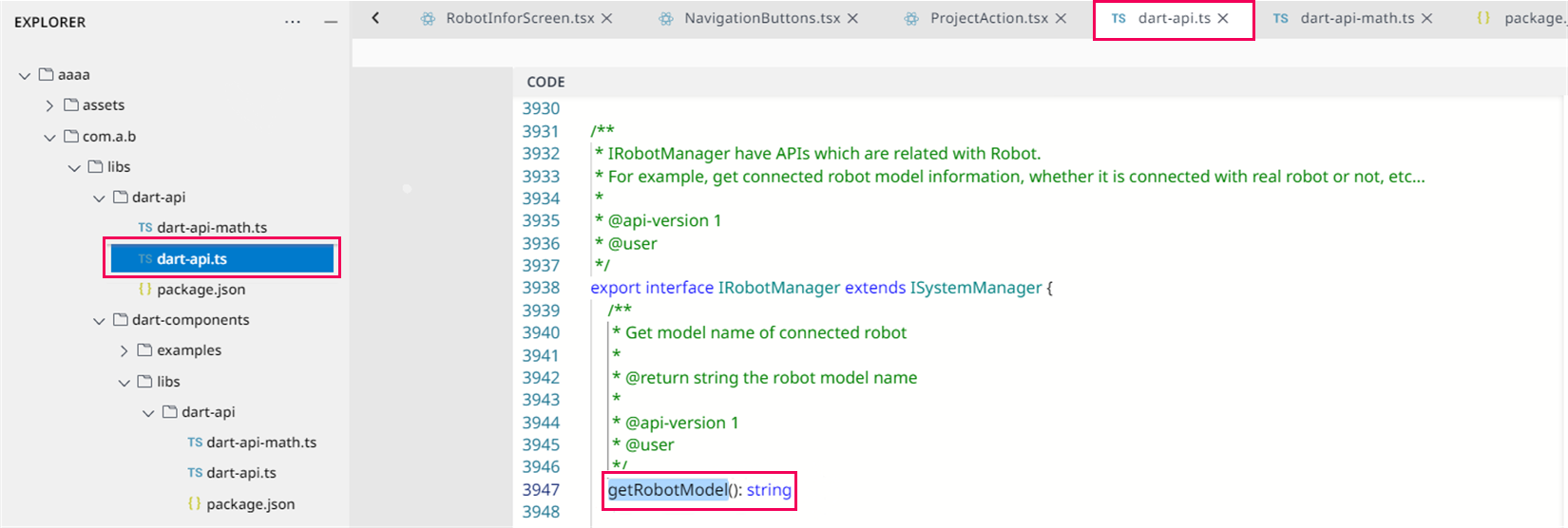
Example for using Dart-API
This is an example of creating three buttons on the screen and calling the API in a different way for each button. This example covers the following UI components and Dart-API.
종류 | 요소 | 설명 |
---|---|---|
UI Component |
| A UI component for showing text. |
| A UI component for using button event. | |
Dart-API |
| Get model name of the connected robot
|
| Get Current Pose
|
The examples are shown as following in Dart-IDE and Dart-Platform.
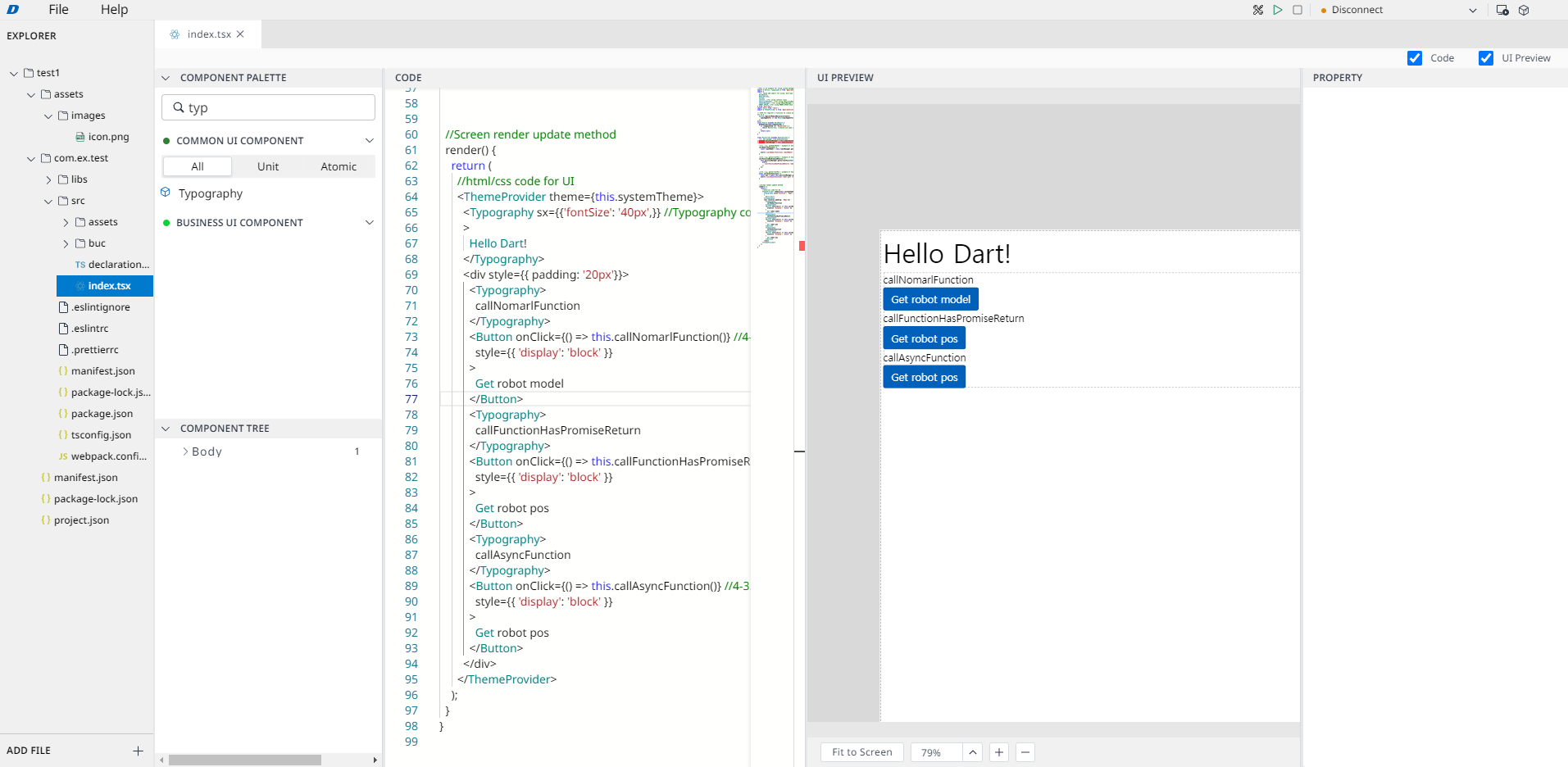
Example in Dart-IDE
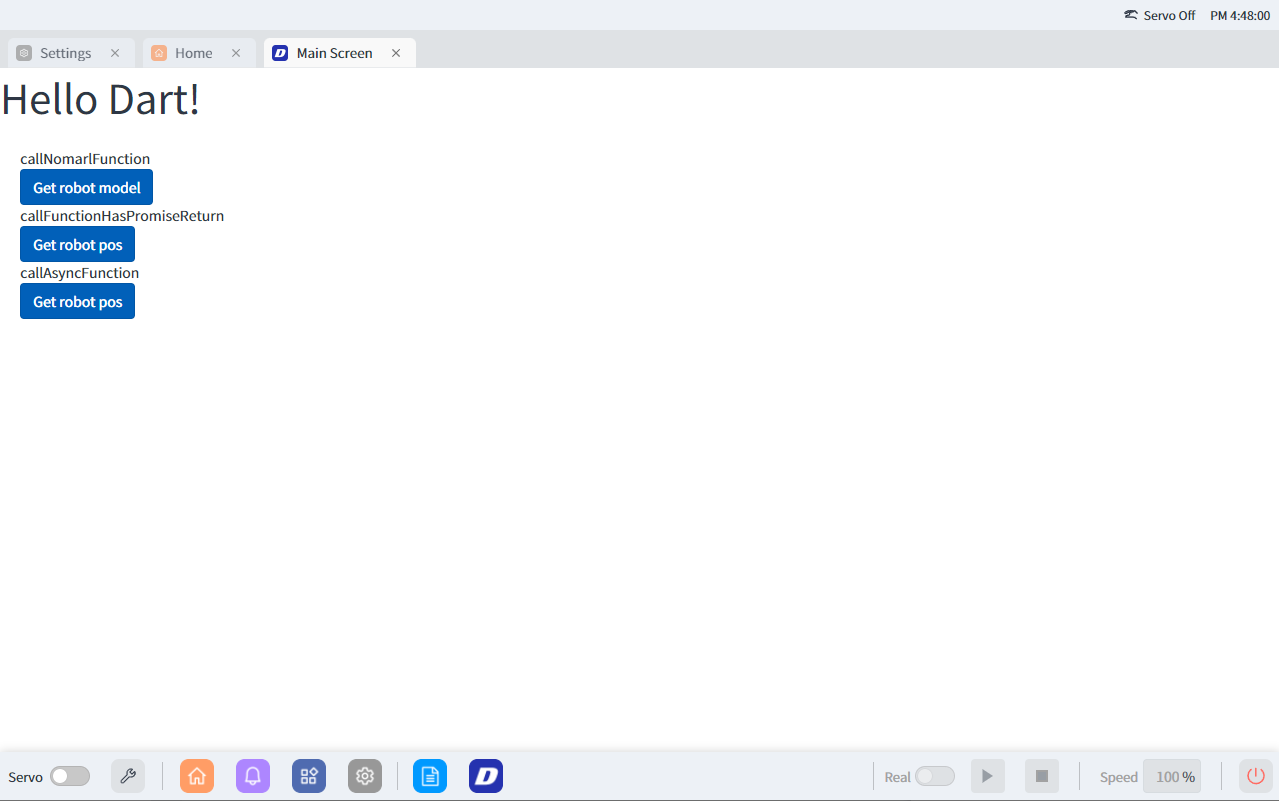
Example in Dart-Platform
The code for this example is as following. Enter the code in the order below in Dart-IDE.
Add import for using 'dart-api' or other from another file.
For more information : https://www.javascripttutorial.net/es-next/javascript-import/
Use same class name below
Get manager from moduleContext
Dart-API examples
e.g. getRobotModel - example of method with normal return
e.g. getCurrentPos - example of how to use promise/then
e.g. getCurrentPos - example of how to use async/await
Add OnClick event on button UI
//This is an example for using system manager and position manager in Dart-API
import { Button, Typography } from '@mui/material';
import {
//1. shoud add import for using 'dart-api' or other from another file.
BaseModule,
ModuleScreen,
System,
Context, //for using context class
IPositionManager, //for using IPositionManager interface.
IRobotManager, //for using IRobotManager interface.
ROBOT_SPACE, //for using ROBOT_SPACE enum type constant.
} from 'dart-api';
import React from 'react';
import { ThemeProvider } from '@mui/material/styles';
// IIFE for register a function to create an instance of main class which is inherited BaseModule.
(() => {
System.registerModuleMainClassCreator(
(packageInfo) => new Module(packageInfo),
);
})();
class Module extends BaseModule {
getModuleScreen(componentId) {
if (componentId === 'MainScreen') {
return MainScreen; //2. should use same class name below
}
return null;
}
}
class MainScreen extends ModuleScreen {
//3. Get manager from moduleContext
private positionManager = this.moduleContext.getSystemManager(Context.POSITION_MANAGER) as IPositionManager;
private robotManager = this.moduleContext.getSystemManager(Context.ROBOT_MANAGER) as IRobotManager;
//4-1. e.g. getRobotModel - example of method with normal return
callNomarlFunction() {
const robotModel = this.robotManager.getRobotModel();
alert(`[callNomarlFunction] robotModel? ${robotModel}`);
}
//4-2. e.g. getCurrentPos - example of how to use promise/then
callFunctionHasPromiseReturn() {
this.positionManager.getCurrentPos(ROBOT_SPACE.JOINT).then((pos) => {
alert(
`[callFunctionHasPromiseReturn] robot pos? \n${JSON.stringify(pos)}`,
);
});
}
//4-3. e.g. getCurrentPos - example of how to use async/await
async callAsyncFunction() {
const pos = await this.positionManager.getCurrentPos(ROBOT_SPACE.JOINT);
alert(`[callAsyncFunction] robot pos? \n${JSON.stringify(pos)}`);
}
//Screen render update method
render() {
return (
//html/css code for UI
<ThemeProvider theme={this.systemTheme}>
<Typography sx={{'fontSize': '40px',}} //Typography component for text UI
>
Hello Dart!
</Typography>
<div style={{ padding: '20px'}}>
<Typography>
callNomarlFunction
</Typography>
<Button onClick={() => this.callNomarlFunction()} //5-1. add OnClick event on button
style={{ 'display': 'block' }}
>
Get robot model
</Button>
<Typography>
callFunctionHasPromiseReturn
</Typography>
<Button onClick={() => this.callFunctionHasPromiseReturn()} //5-2. add OnClick event on button
style={{ 'display': 'block' }}
>
Get robot pos
</Button>
<Typography>
callAsyncFunction
</Typography>
<Button onClick={() => this.callAsyncFunction()} //5-3. add OnClick event on button
style={{ 'display': 'block' }}
>
Get robot pos
</Button>
</div>
</ThemeProvider>
);
}
}
Examples
Example 1. DRL program executable code
class MainScreen extends ModuleScreen {
runDrlProgram (drlAsString: string) {
const programManager = this.moduleContext.getSystemManager(Context.PROGRAM_MANAGER) as IProgramManager;
programManager.runProgram(drlAsString, null, null, null)
.then(result => {
if (result) {
logger.info("Successfully run program.");
} else {
logger.warn(`Failed to run program.`);
}
})
.catch((e: Error) => {
logger.warn(`Failed to run program by ${e}.`);
});
}
}
Example 2. ServoOn status and monitoring check code via IGeneralManager
class MainScreen extends ModuleScreen {
readonly generalManager: IGeneralManager;
constructor(props: ServoSwitchProps) {
super(props);
this.generalManager = this.moduleContext.getSystemManager(Context.GENERAL_MANAGER) as IGeneralManager;
}
componentDidMount() {
// register a callback to notify when servo state has been changed.
this.generalManager.servoState.register(this.moduleContext, this.servoStateCallback);
if (this.generalManager.servoState.value) {
// The servo is now on. Do something.
} else {
// ...
}
}
componentWillUnmount() {
// unregister the registered callback on unmount time.
this.generalManager.servoState.unregister(this.moduleContext, this.servoStateCallback);
}
servoStateCallback = (state: boolean) => {
// TODO: Servo state has been changed. Do something.
}
}