User Command Guide
Change Note
Type | Changes | Compatible Task Editor Module Version | |
---|---|---|---|
1 | NEW | First created. Interface design for ‘User Commands’ function. | v1.0.0
|
2 | NEW |
| v1.4.0
|
3 | REMOVED |
| v1.4.0
|
4 | NEW |
![]() | v2.0.5
|
Overview
Task Editor is a default module included in Dart-Platform and User Command is one of the functions in this module.
1. Interface call relationship diagram
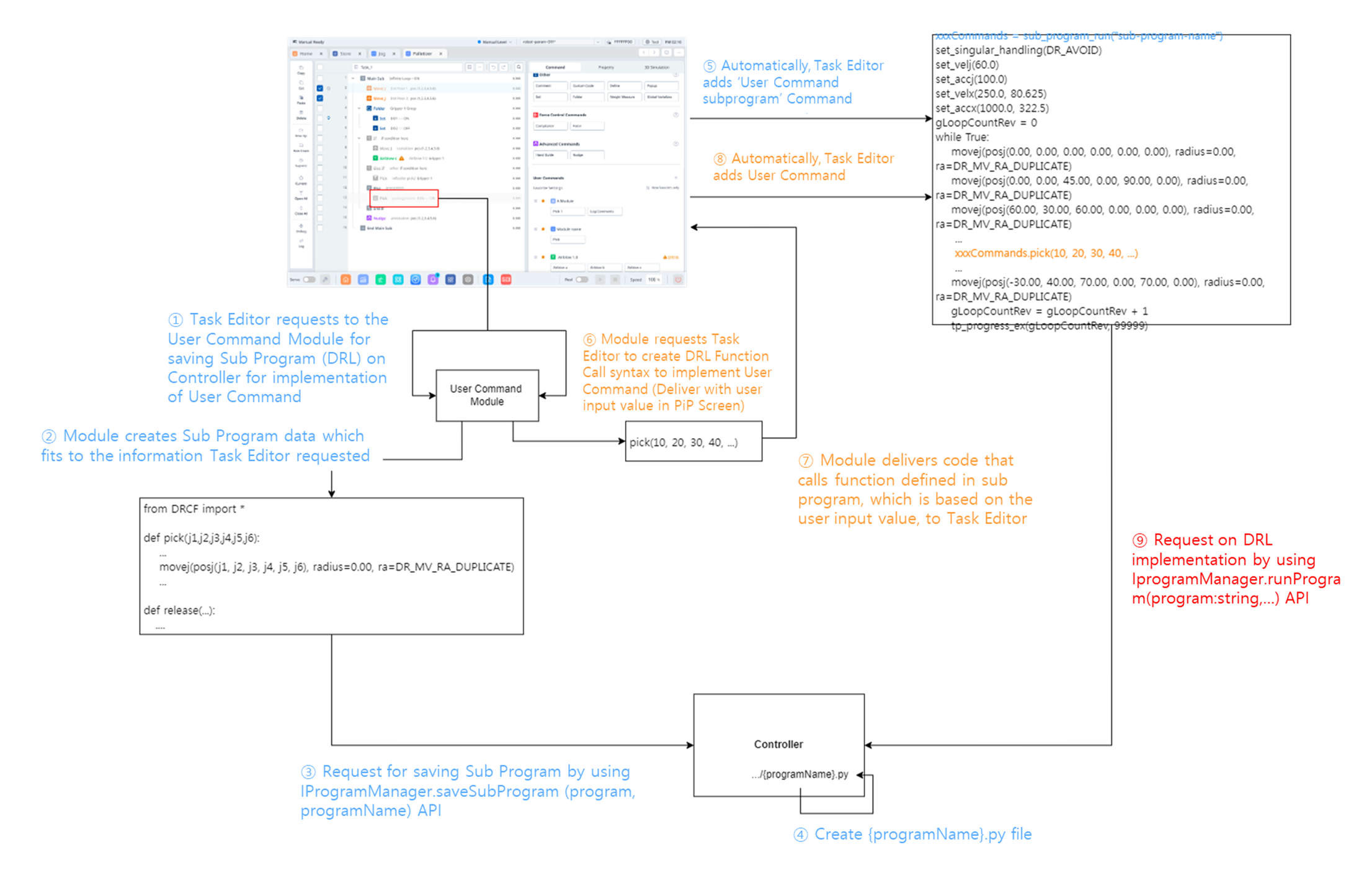
The Interface that User Command type module supports are following below.
Use Case | Description | |
---|---|---|
1 | List-up |
|
2 | Start a PiP Screen with/without saved data |
|
3 | Get data from the PiP Screen |
|
4 | Request save DRL Function Definition for the User Command to the Controller |
CODE
|
5 | Generate Function Call of the User Command |
CODE
|
2. Detailed Definition on the Interface and its Example codes
Task Editor module considers defined component ‘com.dart.module.taskeditor.action.USER_COMMAND’ as module component that provides interface for ‘User Command’.
2-1) List-up
When entering into Task writing screen of Task Editor module.
Purpose : To display User Command module block which is installed in Dart-Platform within the ‘User Commands’ Category.
Method : IModulePackageManager & Message
Name | Value |
---|---|
action |
|
category |
|
2-2) Start a PiP Screen with/without saved data
When selecting ‘User Command’ block which is added within the task of Task Editor module
Purpose: To displaying the PRoperty of selected User Command (PiP Screen) and initialize data.
Method : IModuleScreenManager & Message
Sort | Value | ||
---|---|---|---|
action |
| ||
category |
| ||
componentId |
| ||
data | key | type | notice |
savedData | Record<string, any> | undefined |
| |
savedVersion | string |
|
2-3) Get data from PiP Screen
When saving the task of Task Editor module
Purpose: To transmit and receive data in order to save the user’s entered value within Property (PiP Screen) of User command module into Task Editor DB.
Method: ModuleScreen & IModuleChannel
Sort | Value | ||
---|---|---|---|
eventName | arguments | notice | |
send (request) | get_current_data | - |
|
receive (response) | get_current_data | Record<string, any> | undefined |
|
2-4) Request to save Commands Definition as sub program / Generate Command Call through ModuleService
When generating DRL syntax for task execution in Task Editor module
Purpose : To transmit and receive the DRL syntax information on User Command used in Task.
Method : ModuleService & IModuleChannel
Sort | Value | ||
---|---|---|---|
eventName | arguments | notice | |
send (request) | req_to_save_commands_def_as_sub_program | { programName: string } |
|
receive (response) | req_to_save_commands_def_as_sub_program | boolean |
|
send (request) | gen_command_call |
TYPESCRIPT
|
|
receive (response) | gen_command_call | string |
|
e.g. default return value
CODE
e.g. When the data is invalid and the DRL cannot be generated, the value should be provided as shown below.
CODE
An example DRL that is generated is as follows:
CODE
| |||
TYPESCRIPT
|
2-5) Get / Watch Global / System Variables from Task Editor
When selecting the ‘User Command’ block added within the task of the Task Editor module
Purpose: Provide variables to store result values in the Property (PiP Screen) of the selected User Command
Method: IModuleScreenManager & Message
This API is the API that the Task Editor module must provide for the User Command module.
Sort | Value | ||
---|---|---|---|
eventName | arguments | notice | |
send (request) | get_variables | - |
|
receive (response) | get_variables | { name: string division: 0|1 type: 0|1|2|3|4|5|6|7 }[] |
CODE
|
send (request) | changed_variables | { name: string division: 0|1 type: 0|1|2|3|4|5|6|7 }[] |
|
receive (response) | changed_variables | { name: string division: 0|1 type: 0|1|2|3|4|5|6|7 }[] |
|