Language Resources
Because the react-i18next library is used in the Dart-Platform, you can support multiple languages on your module by using the same i18next method. Then the multiple languages will be supported within the Dart-Platform.
Info.
i18next is internationalized framework written with JavaScript which allows handling of multiple national languages.
You can find
index.tsx
file in EXPLORER windowProject name
▸Package name
▸src
▸assets
▸langs
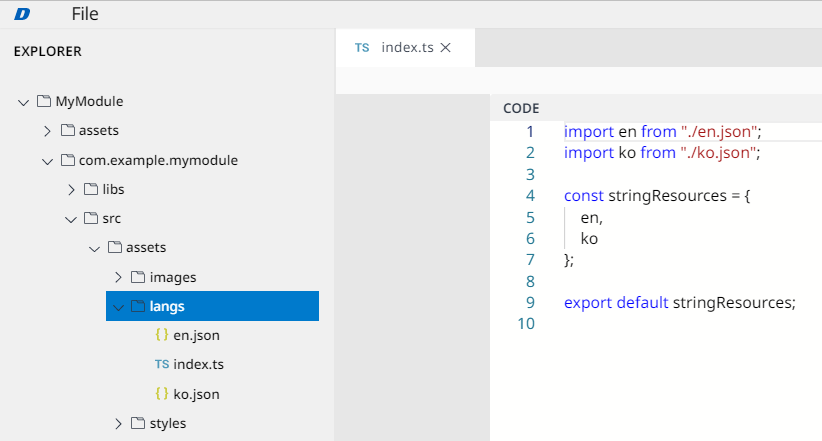
Use language resources in index.tsx
Registering multilingual is simple.
Step 1. Define the language to use in this module within the
index.ts
file.
index.ts
is a file for multilingual resource file (json) that exists under langs. Define and export stringResources as follows.
import en from "./en.json";
import ko from "./ko.json";
const stringResources = {
en,
ko
};
export default stringResources;
Currently Dart-Platform officially supports 11 languages as following (expected).
import ko from "./ko.json";
import en from "./en.json";
import nl from "./nl.json";
import it from "./it.json";
import zh from "./zh.json";
import de from "./de.json";
import fr from "./fr.json";
import es from "./es.json";
import ja from "./ja.json";
import cs from "./cs.json";
import hu from "./hu.json";
const stringResources = {
en,
ko,
nl,
it,
zh,
de,
fr,
es,
ja,
cs,
hu,
};
export default stringResources;
Step 2. Create
{language_code-REGION}.json
each inlangs
folder and define resource of each language by key:value pair.For example the file name can be en.json, and ko.json.
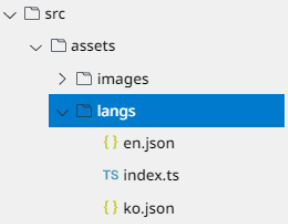
{
//module package name
"{app_package_name}": {
//"key": "value"
"example_key" : "Example Value",
"app_name" : "test module",
}
}
Step 3. There are two following ways to support multilingual in
index.tsx
.Method 1. Function Type React component
Method 2. Class type React component
Method 1. Use in Function type React component
Import library
import { useTranslation } from "react-i18next";
Use Translation Function
const { t } = useTranslation();
{t("app_name", { ns: packageName })}
// index.tsx
import { ThemeProvider } from "@mui/material/styles";
import { useTranslation } from "react-i18next";
...
class MainScreen extends ModuleScreen {
...
render(){
return(
<ThemeProvider theme={this.systemTheme}>
<AppName/>
</ThemeProvider>
)
}
}
function AppName(props: { moduleContext: ModuleContext }) {
const { moduleContext } = props;
const { packageName } = moduleContext;
const { t } = useTranslation();
return (
<div>
<h3>{t("app_name", { ns: packageName })}</h3>// Use Module's language resource
<h3>{t("app_name")}</h3> // Use System (Dart-Platform)'s resources
</div>
);
}
Method 2. Use in Class type React component
// index.tsx
import { ThemeProvider } from "@mui/material/styles";
import { Translation } from "react-i18next";
...
class MainScreen extends ModuleScreen {
...
render(){
return(
<ThemeProvider theme={this.systemTheme}>
<Translation>
t =>
<section className={styles["container_servo-switch"]}>
<h3>{t("app_name", { ns: this.moduleContext.packageName })}</h3> // Use App's language resource
<h3>{t("app_name")}</h3> // Use System (Dart-Platform)'s resources
</section>
<Translation>
</ThemeProvider>
)
}
}