Develop User Command
Overview
User Command refers to various commands that operate on the robot. User Command operates in the Task Editor module that is installed by default in the Dart-Platform, and can create a number of commands necessary for robot operation, such as basic motion, communication, and control of the robot.
User Command can be operated using DRL, and can not only be used independently in the Task Editor, but can also be used in conjunction with the installed module of the Dart-Platform.
1. How to make User Command
Make New Project
Create a new project and select User Command as shown below to create the project.
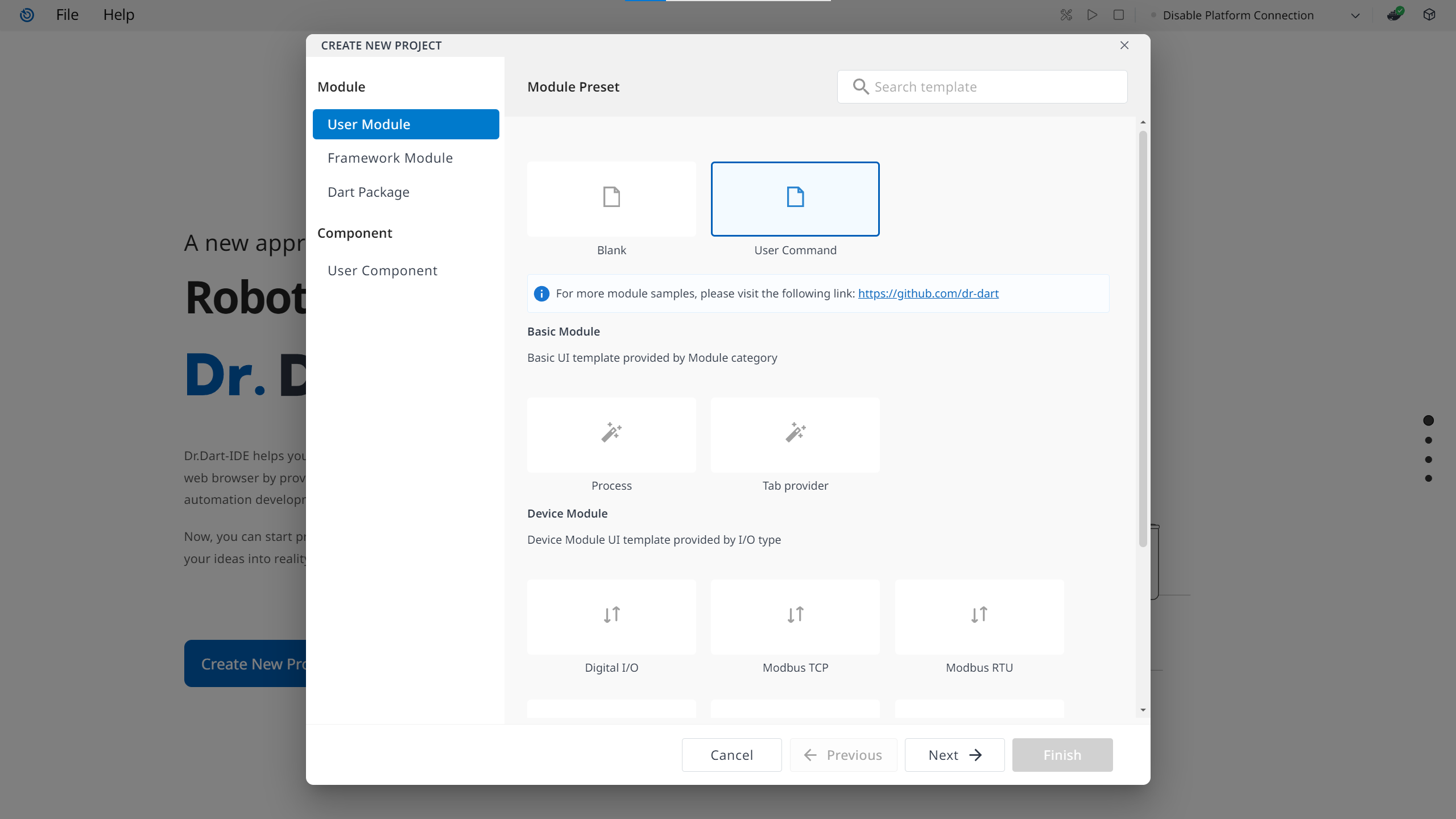
Define Command Name
You can add a command name by writing code directly, but you can add a command more easily using Visual Scripting.
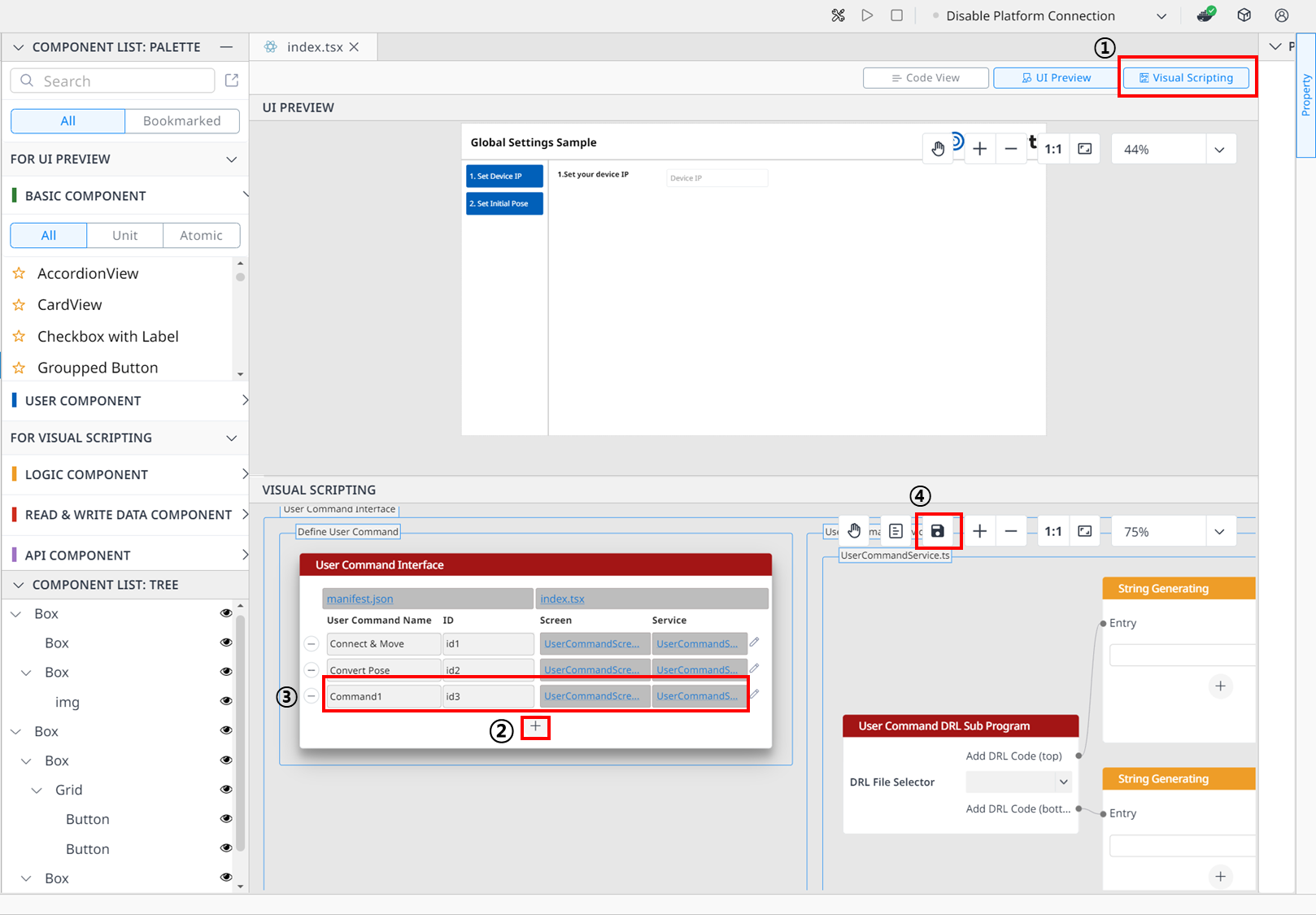
Press the “+” button in the “User Command Interface” Node, then enter the command name and ID (unique ID) you want to add.
Note: After setting the Command name, you must click the Save button to save the changes.
If an error occurs in Main Screen Ui Preview after adding the command, add the following code in UserCommandService.ts below.
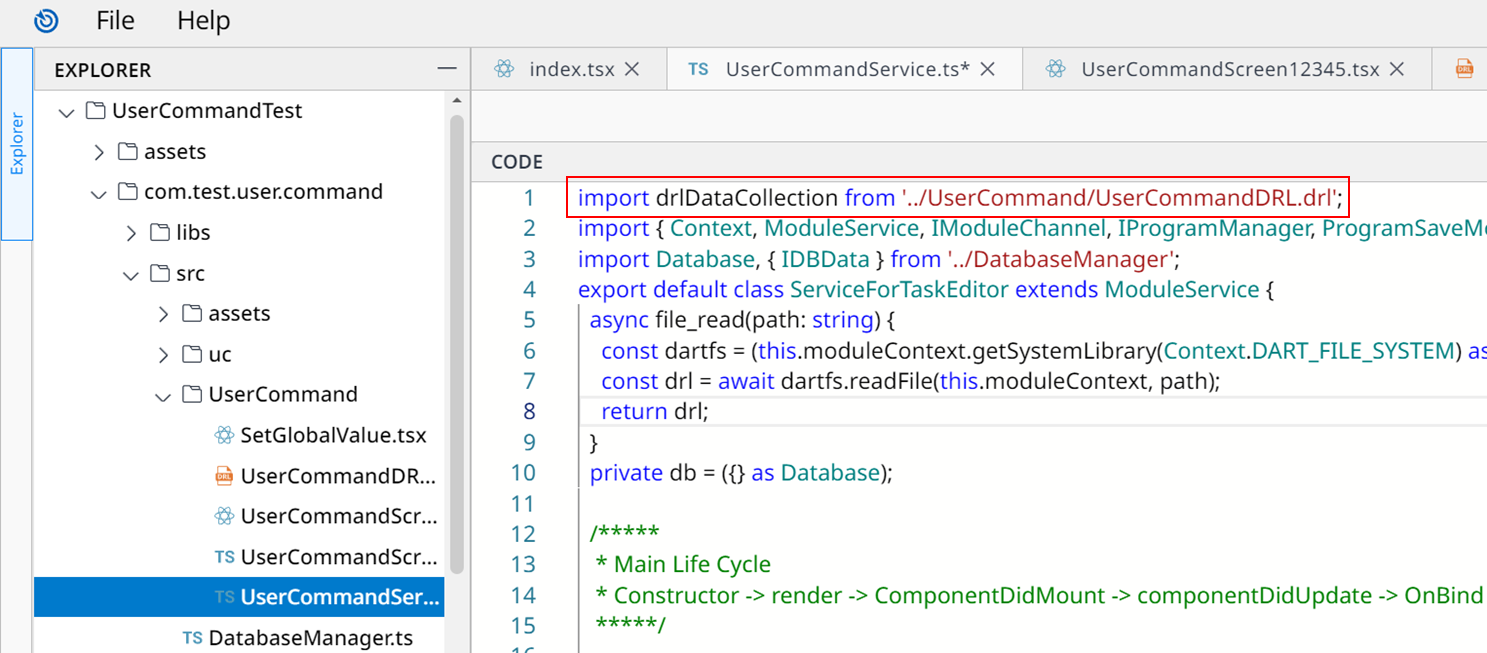
After saving the changes, the Command settings are automatically added to the “<packageName>/manifest.json” file, the UI Screen related to the Command, and the functions required to execute the Command are added.
// manifest.json
"screens": [
{
"name": "Command1",
"id": "id3",
"messageFilters": [
{
"action": "com.dart.module.taskeditor.action.USER_COMMAND",
"category": "dart.message.category.PIP_SCREEN"
}
]
}
]
......
"services": [
{
"name": "Command1",
"id": "id3",
"messageFilters": [
{
"action": "com.dart.module.taskeditor.action.USER_COMMAND",
"category": "dart.message.category.SERVICE"
}
]
}
]
Define DRL function
To create a User Command, you must write a DRL. Before writing a DRL, you must register the name of the function you want to add as shown below. The registration method can be written in code, but registration can be done easily using Visual Scripting.
Define DRL Function
Open the UserCommandDRL.drl file in the UserCommand folder and write the function you want to add.
Here, a function was written to display the values entered in arg1, arg2, and arg3 through tp_popup.
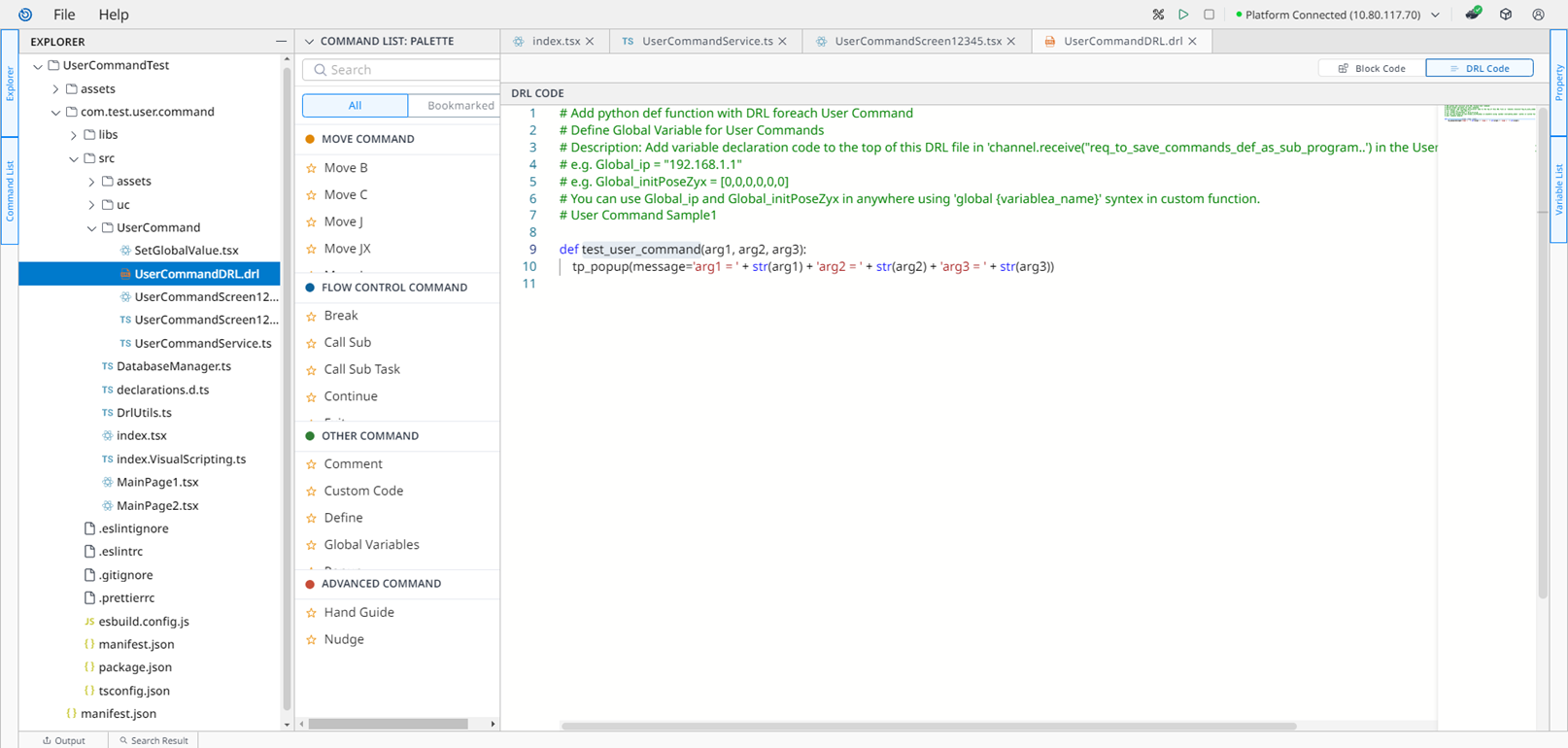
Add Function arguments
Set the arguments applied to the DRL function in Visual Scripting corresponding to the generated Command.
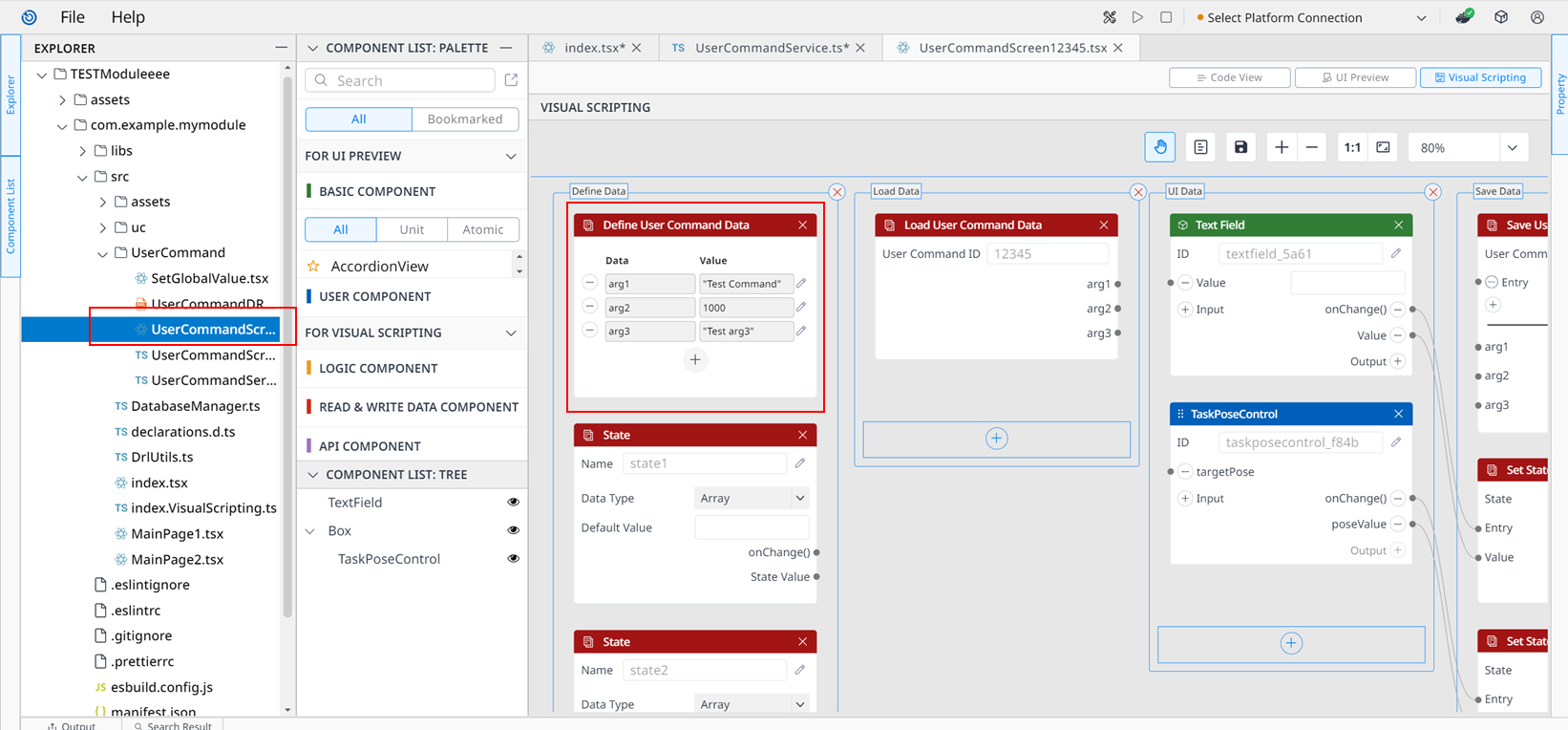
Note: After setting arguments, you must click the Save button.
If the arguments have been created properly, enter the command to be executed in the UserCommand/UserCommandService.ts file in Code as shown below.
// UserCommand/UserCommandService.ts
................
.....
channel.receive('gen_command_call', ({
componentId,
data
}) => {
let result = ``;
// Start describe your function which you will call command
const stringGenerating_12345 = `test_user_command("${data.arg1}",${data.arg2},"${data.arg3}")`;
if (componentId === '12345') {
result += stringGenerating_12345;
}
// End
channel.send('gen_command_call', {
command: result,
variableName: JSON.stringify(data.globalValue) != `{}` ? data.globalValue : ''
});
});
return true;
}
In addition to setting by writing directly in code, the above settings can also be made in the Visual Scripting menu of index.tsx as shown below.
There are several rules to set commands using Visual Scripting.
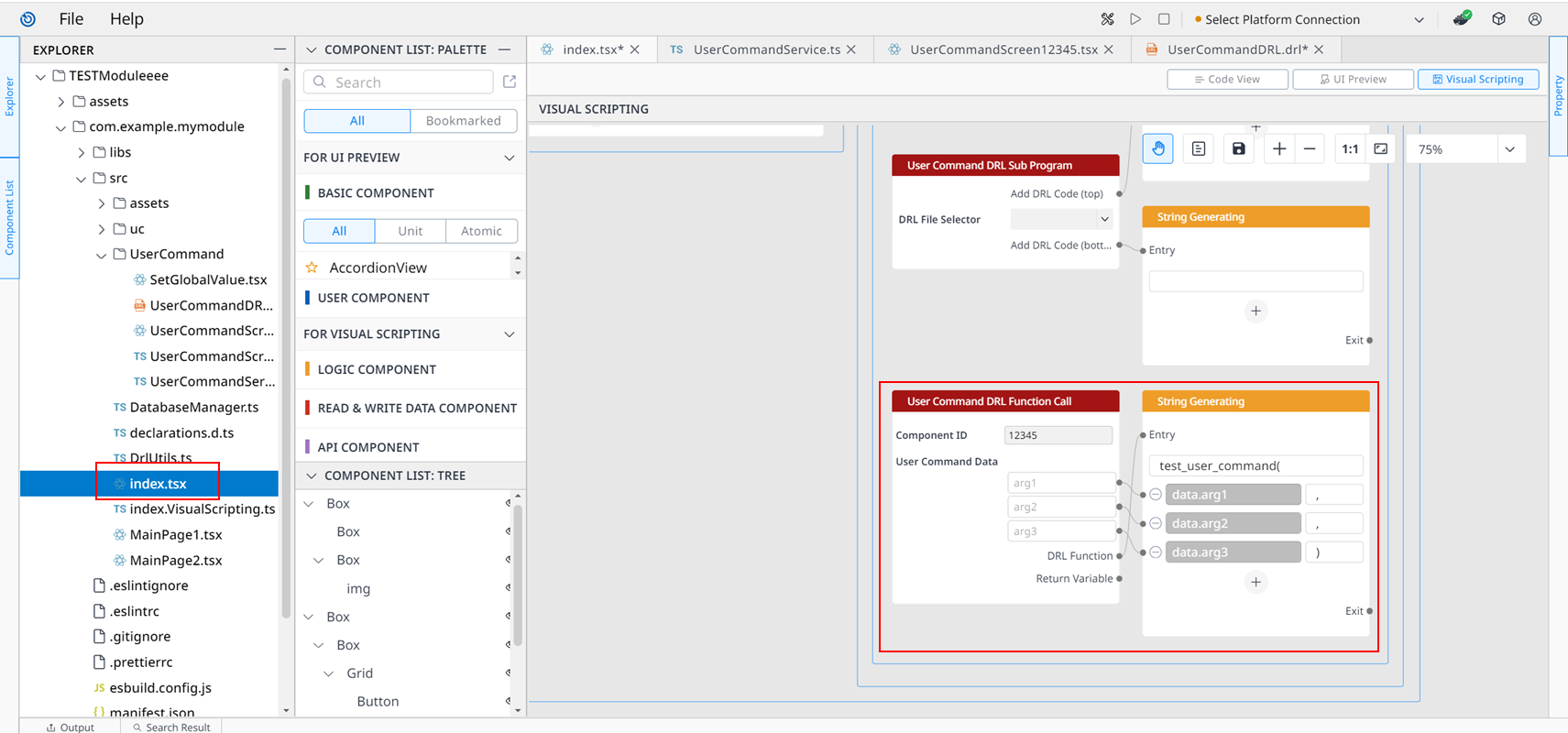
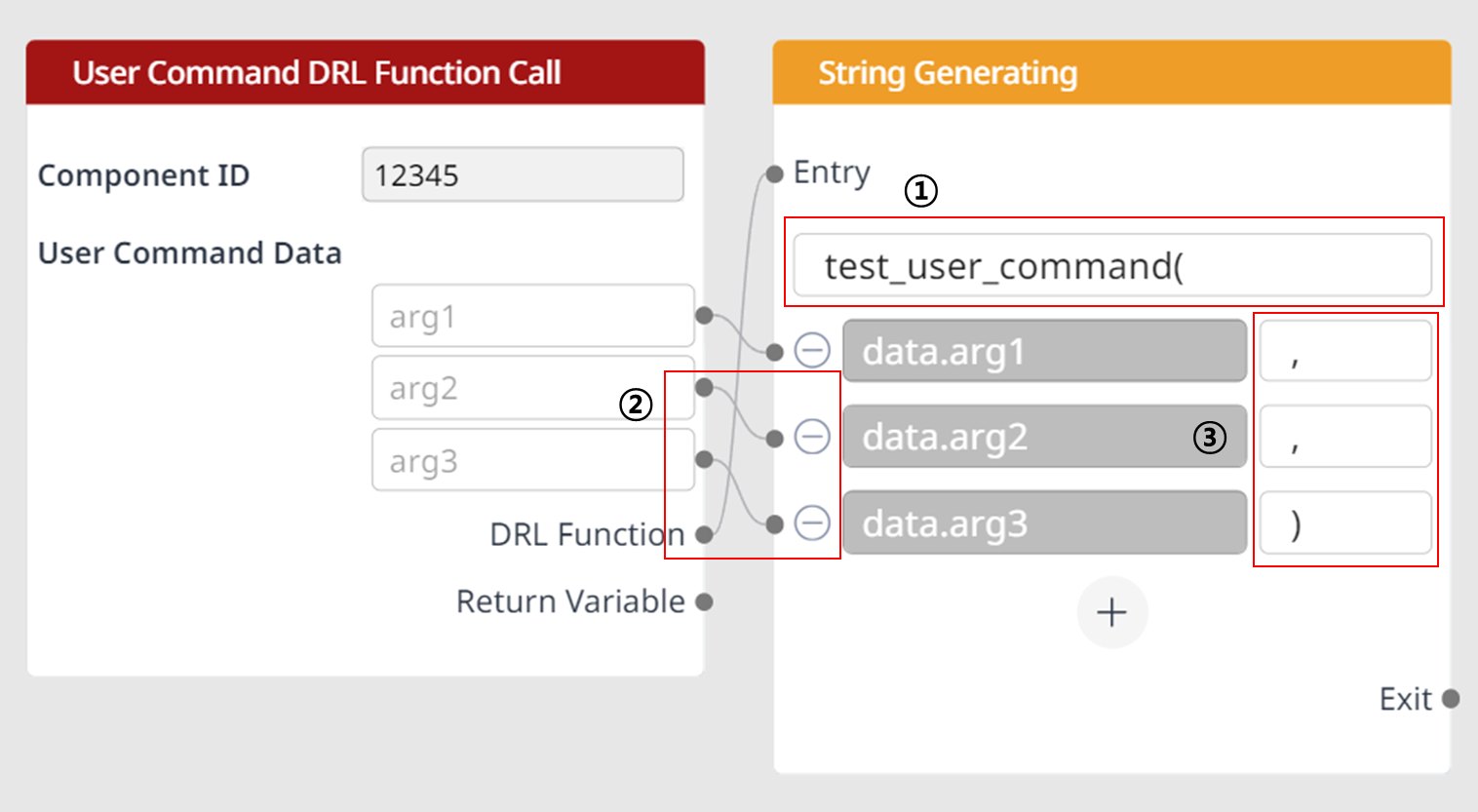
Enter the name of the function you want to run. At the end of the function name, you must include the “(“ symbol, which indicates the beginning of the argument.
Add the argument to be delivered in the “String Generating” Node (click the “+” button) and connect the argument to be delivered in the “User Command DRL Function Call” Node.
You must connect the separators as shown in the picture. If the value is an array, you must enter the “[]” symbols in order as shown in the picture above.
Note: When Argument is set to String and a function is created using VisualScripting, ““ must be added in UserCommandService.ts as follows.

Make UI Property
You must proceed with the process of configuring a configurable UI in the added Command and saving the entered values in DataBase. Additionally, it explains how to implement actions that change the value entered by the user in the UI.
The UI described here will consist of the screens below. This is the screen displayed in TaskEditor, and the screen composition will vary depending on the command.
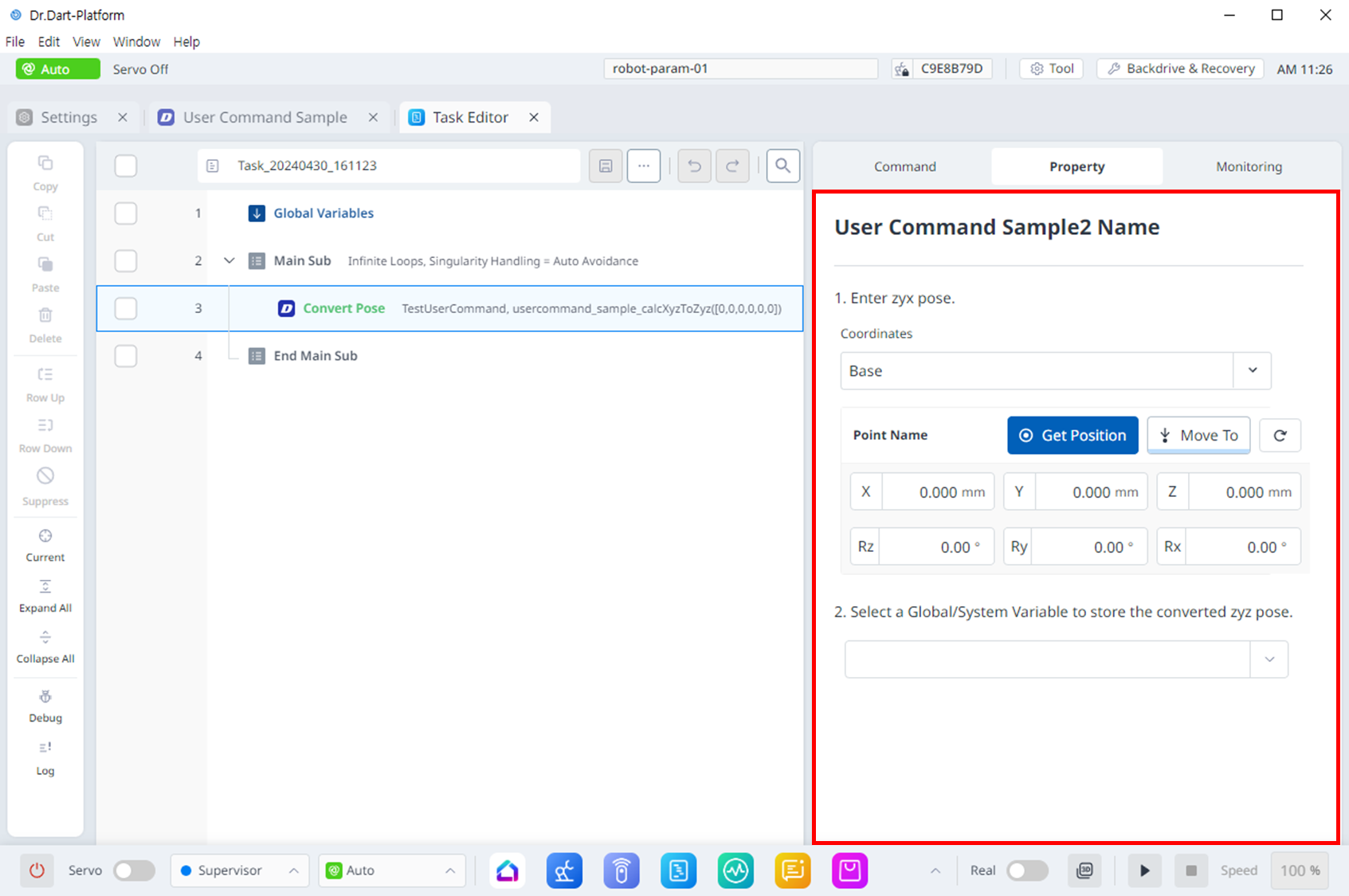
Set UI Property in Visual Scripting
This chapter explains the connection between the composed UI Components and the Argument passed to the Command (DRL) with a simple example.
First, let's delete the UI Components predefined in UI Preview and the UI Data node group in Visual Scripting and add three TextFields.
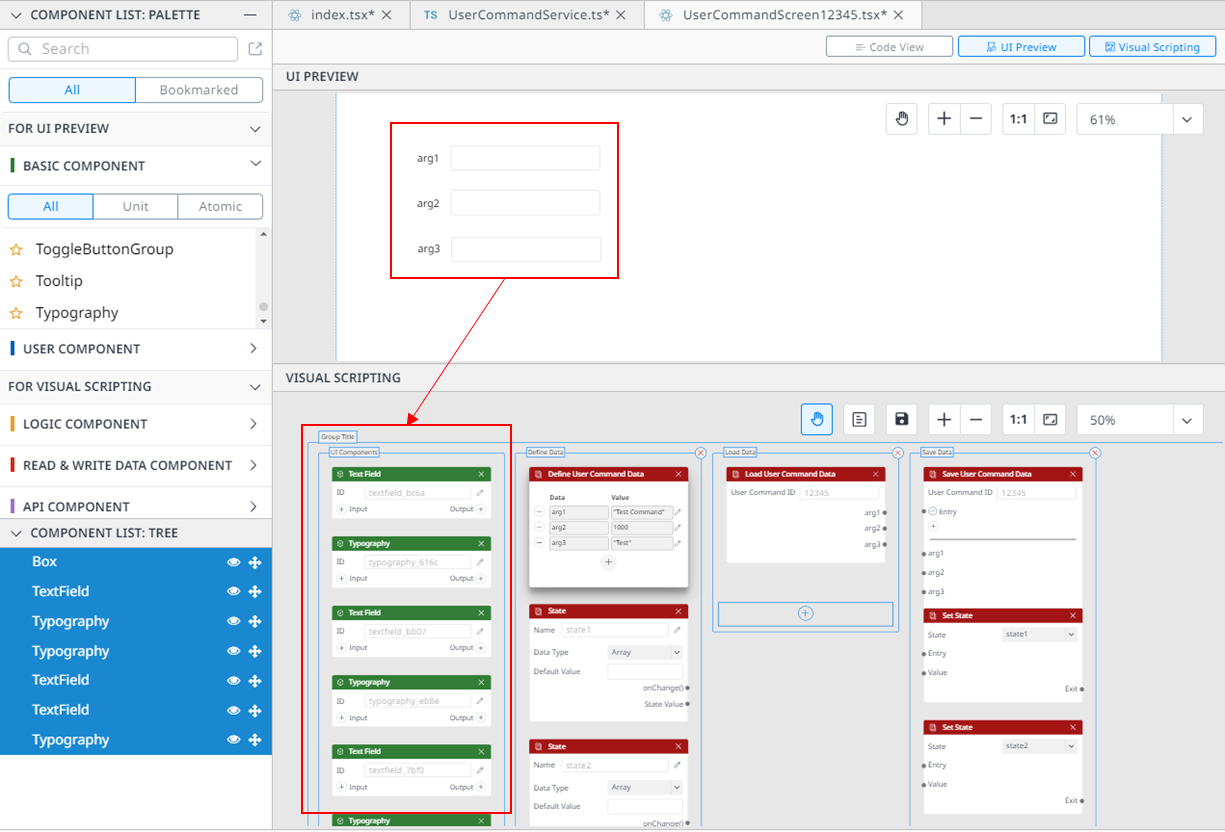
As shown in the picture above, if you add 3 TextFields to UI Preview, you can see that 3 TextField nodes are added to the “UI Components” Node group in Visual Scripting.
The UI Component Node is composed of Input and Output settings. Press the Input button as shown below to select the Value, then specify and connect one of the Arguments entering the DRL displayed in “Load User Command Data” as shown below.
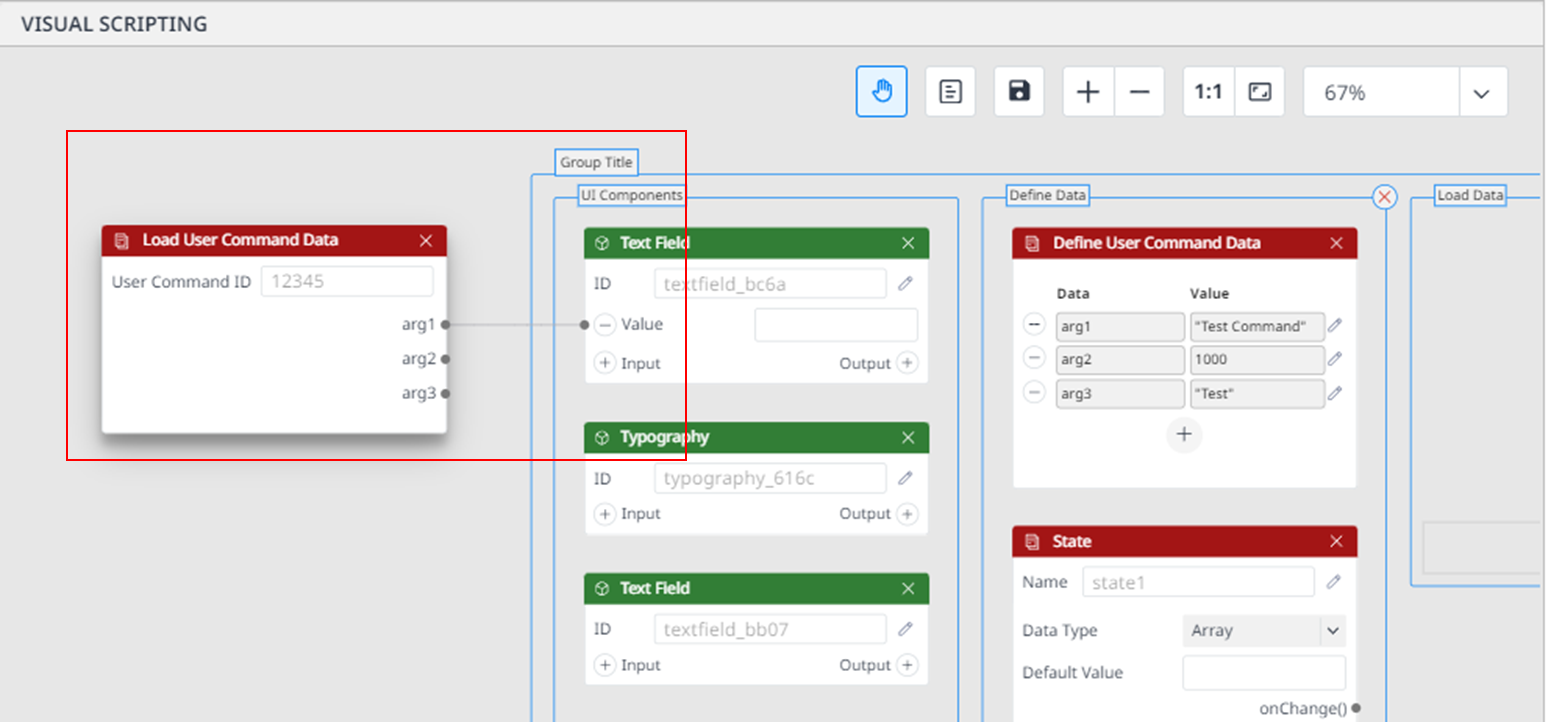
This means that the value entered in the UI is entered as the value of the DRL's Augment (arg1 in the picture above).
Connect the remaining TextFields in the same way.
Note: Node blocks within the Node Group can be freely moved except for the UI Components Node, and if the connection between nodes is complex, the Node location can be changed.
After completing the previous data loading function, this time we will explain the operation of the connection to save the entered value. The Node related to saving data is the “Save User Command Data” Node.
First, press the Output button on the TextField Node. By pressing the Output button, you can select various Event Properties corresponding to TextField. This time, we add two things: onChange() and Value.
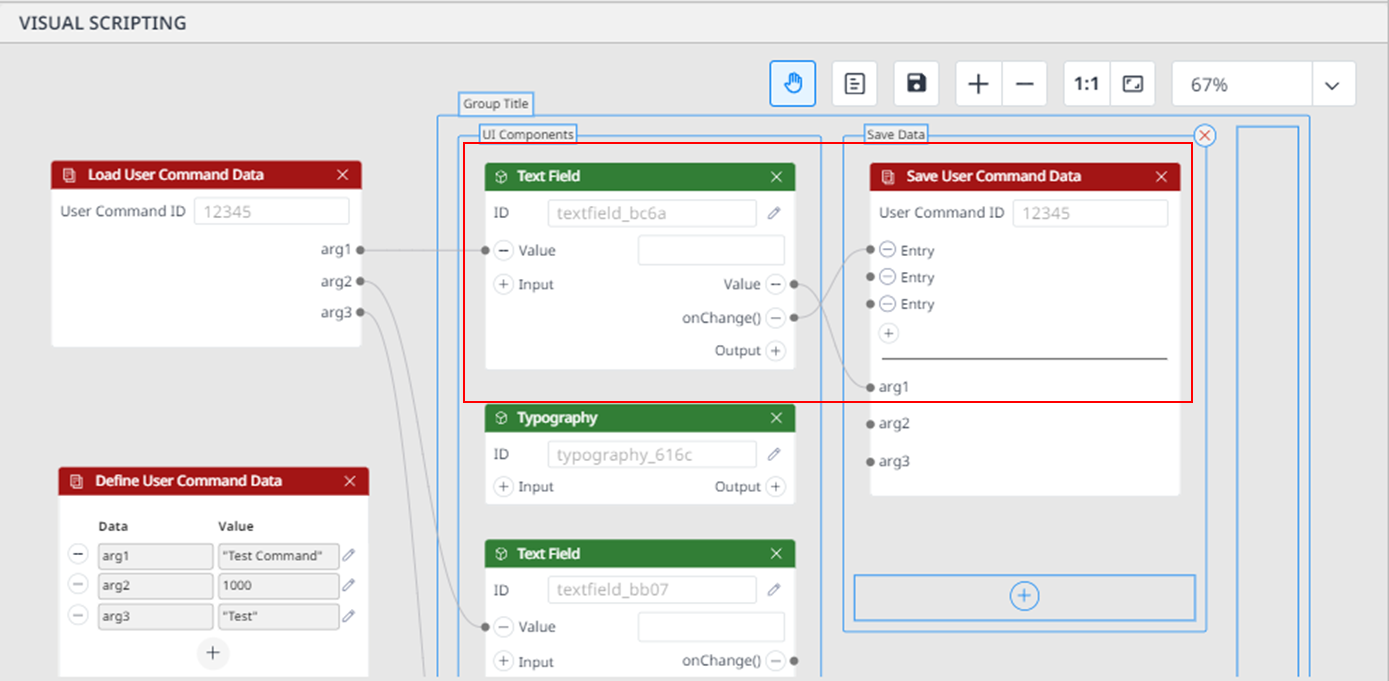
As shown in the picture, connect the added Event (onChange()) to “Entry” of “Save User Command Data”, and connect the Value to the Argument (arg1 in the picture above) of the DRL to be saved. Set the remaining TextField Nodes the same way.
When you complete the settings and install it on the Dart-Platform, a command will appear in the Task Editor as follows.
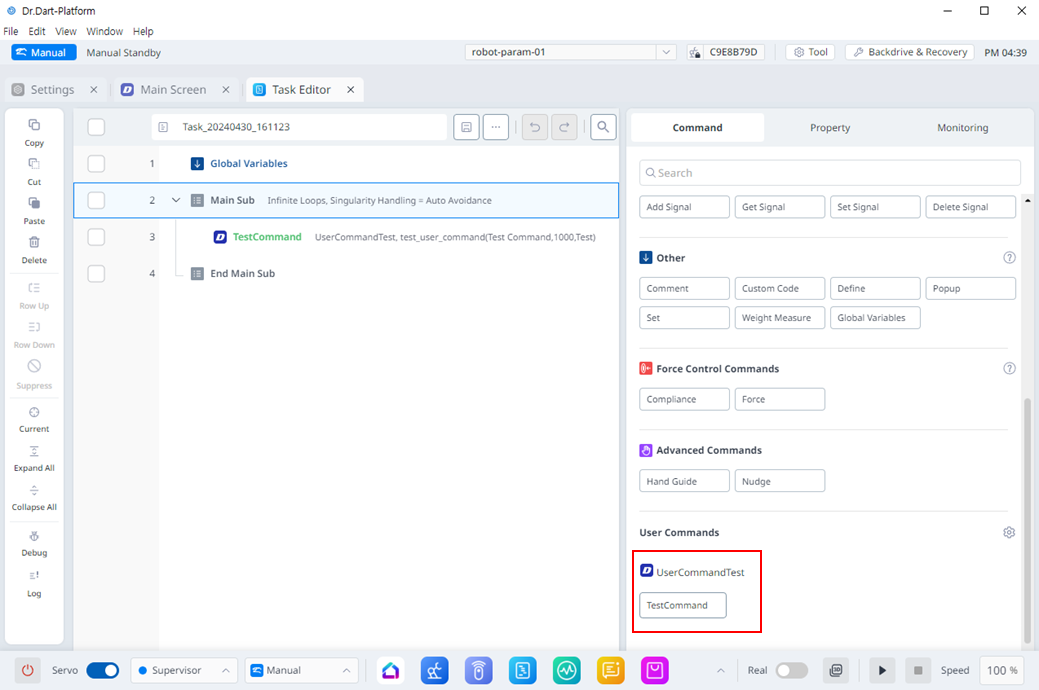
If you add a Command and look at the “Property” Tab, you will see three TextFields appear. And when you run the command, you can see a message appear depending on the value entered in the TextField.
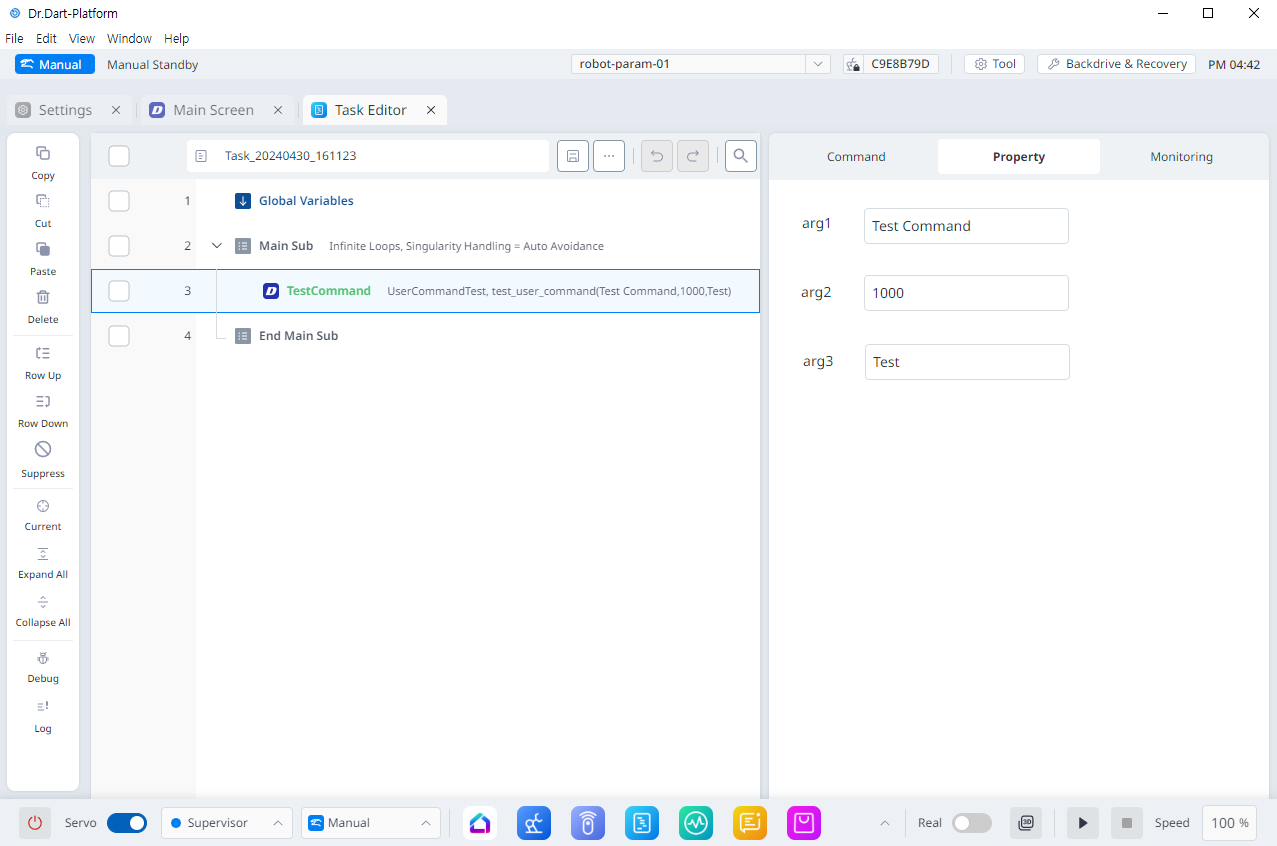
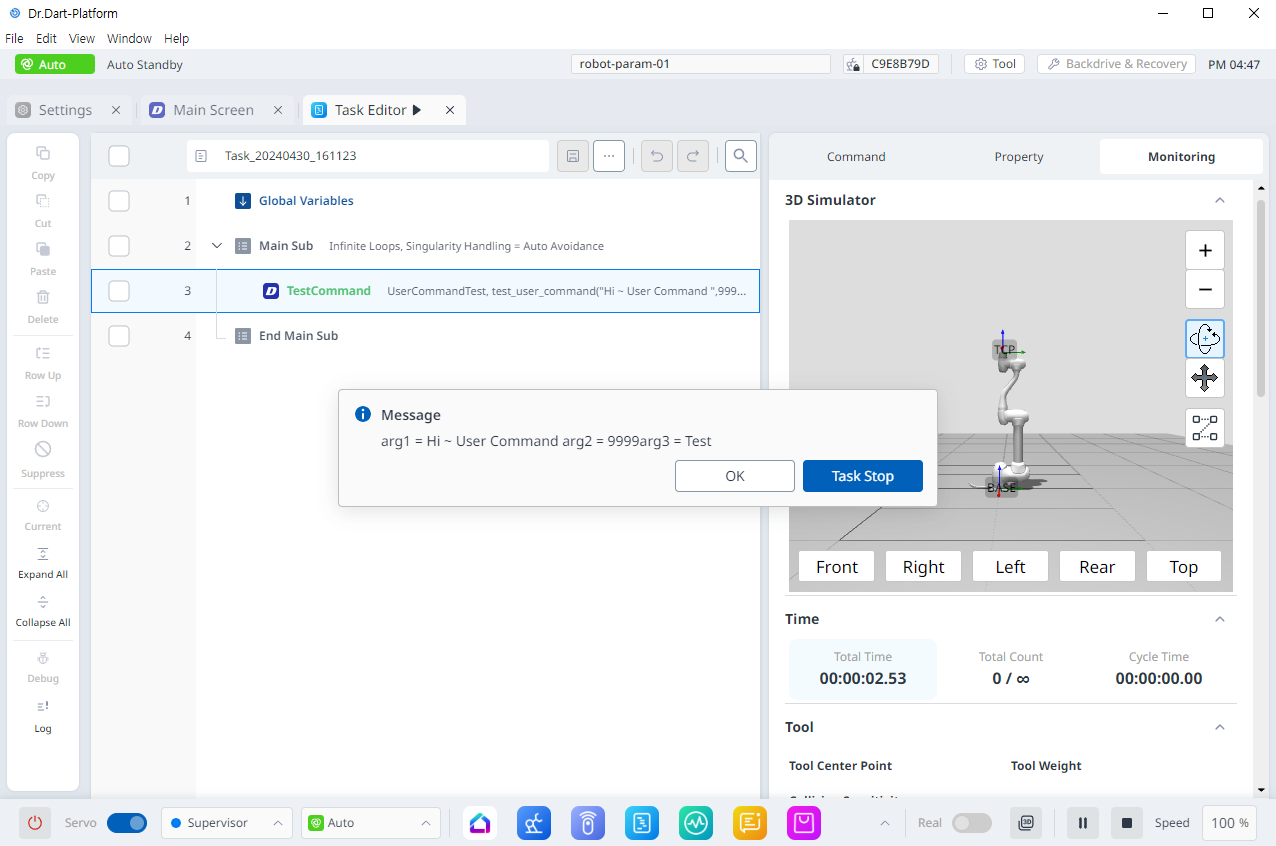
DRL example for input array values
This example explains how to receive array values as input and pass it to the DRL Argument. In this example, we will configure and set up two TextFields to receive input of a single value and array values.
As described above, add two TextFields to the UI Preview as shown below and add two Arguments to the “Define User Command Data” Node.
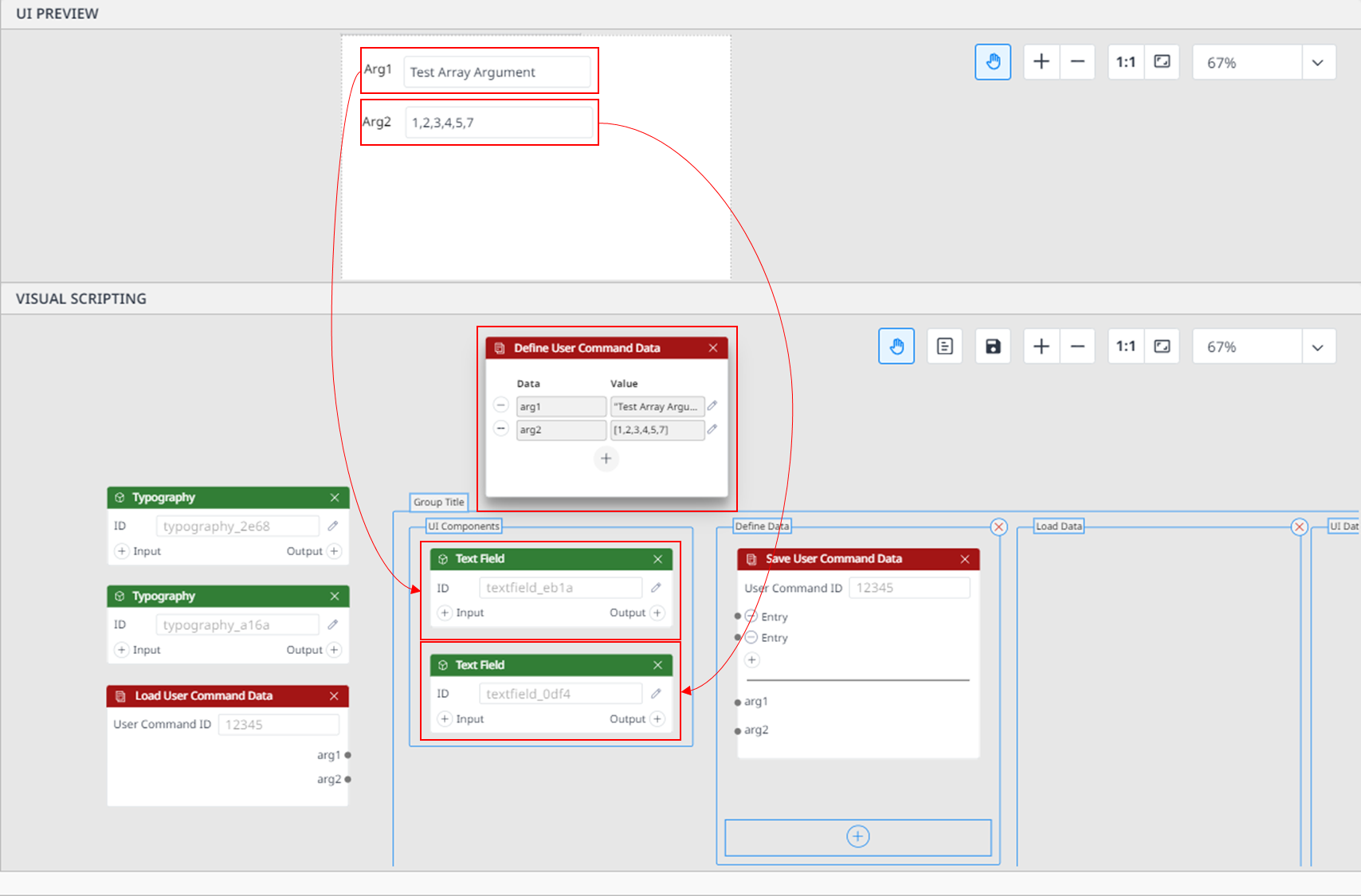
Note: To input array values, you must set the value using the [ ] symbol.
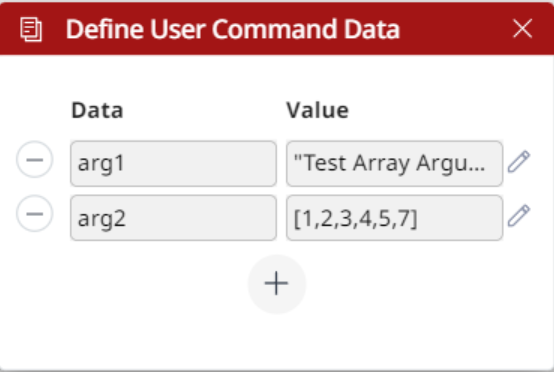
After completing the UI settings, proceed with the connection operation of the “Load User Command Data” Node and the “Save User Command Data” Node as shown below, then save the modified contents.
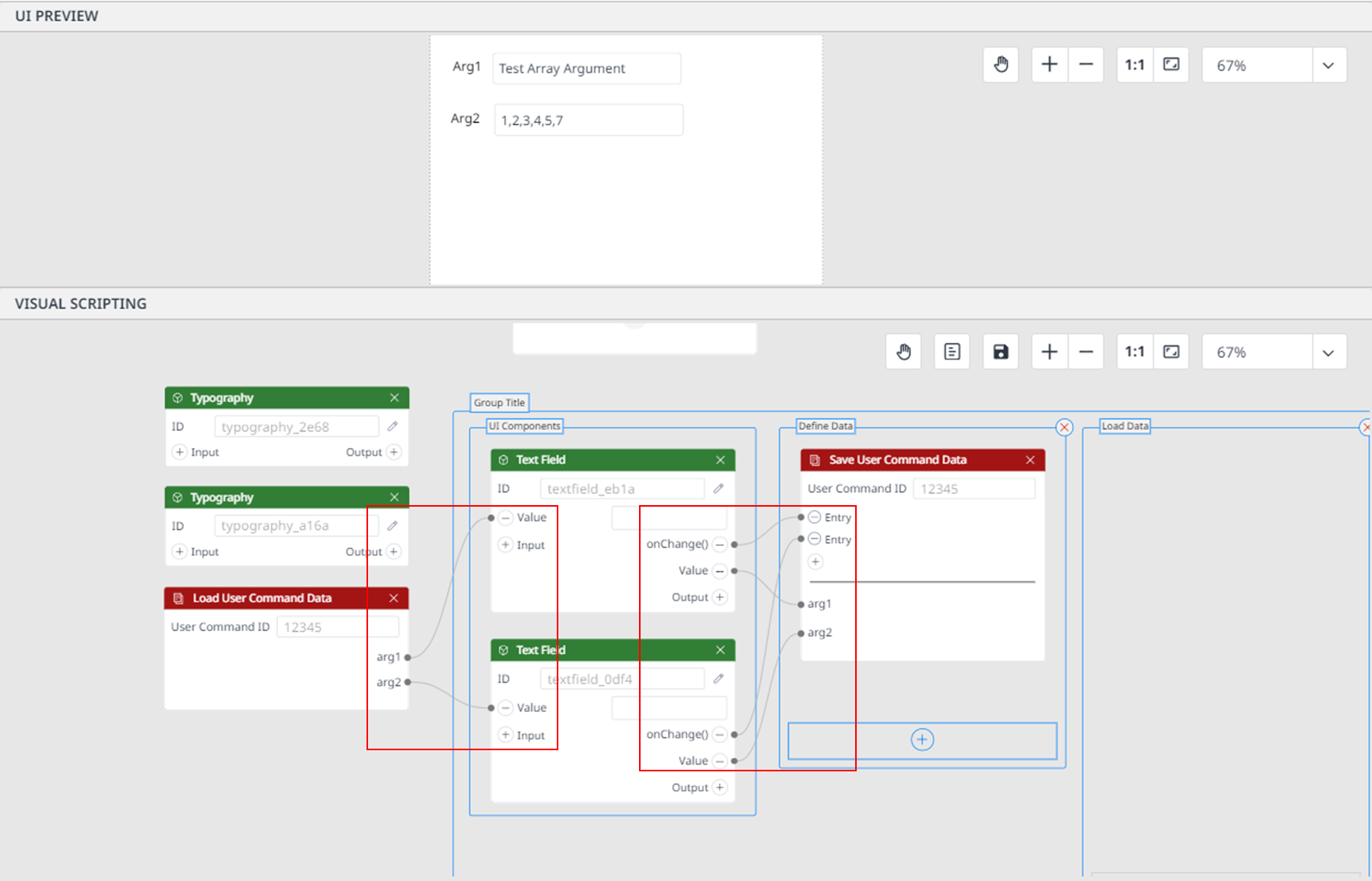
If you have completed the connection setup, now proceed with setting the function to be executed and the Argument in Visual Scripting in index.tsx.
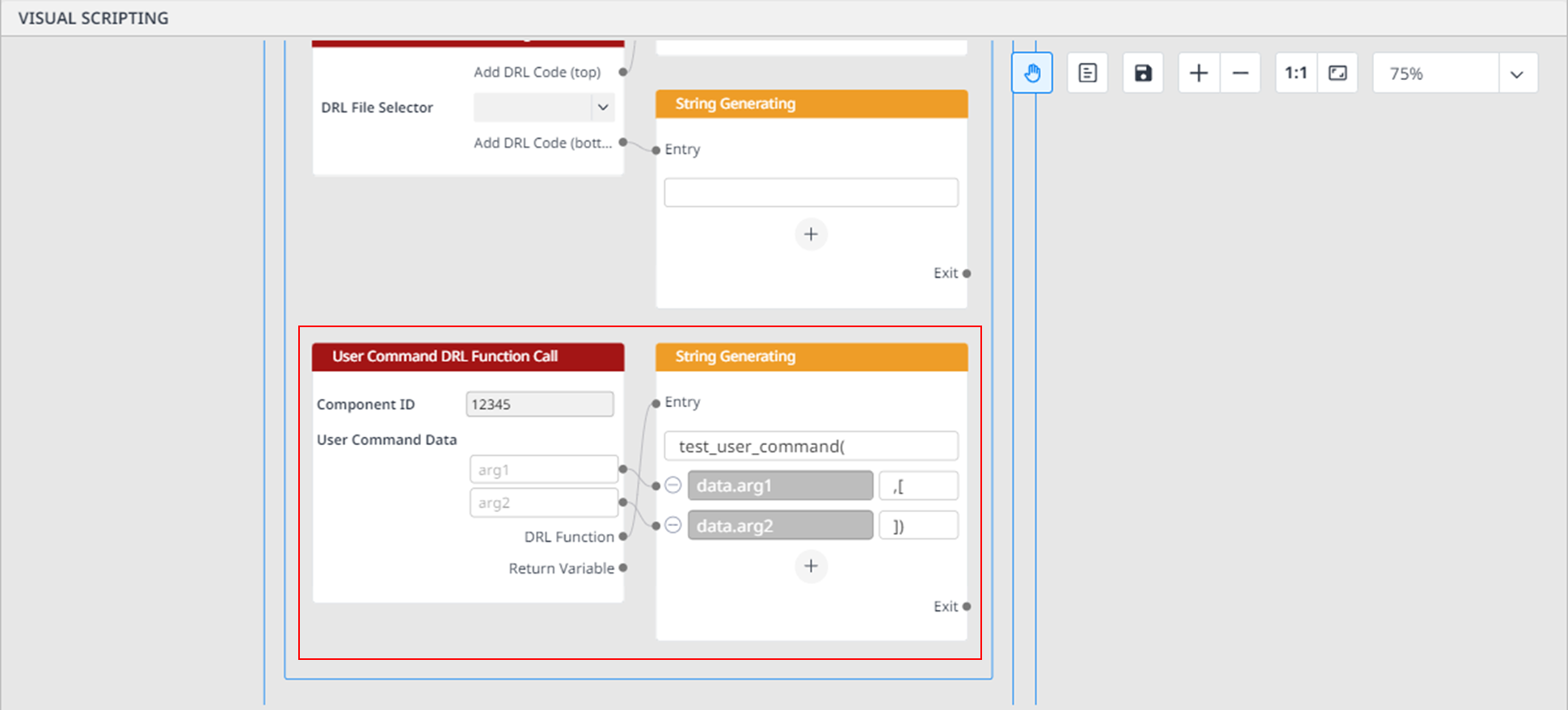
Note: The Argument of this function includes Array, so when setting the Argument in the “String Generating” Node, check that the [] symbol is used as shown above.
When you install the configured Command, a TextField will appear where you can input array values as shown below. When entering, separate the values using the “,” symbol.
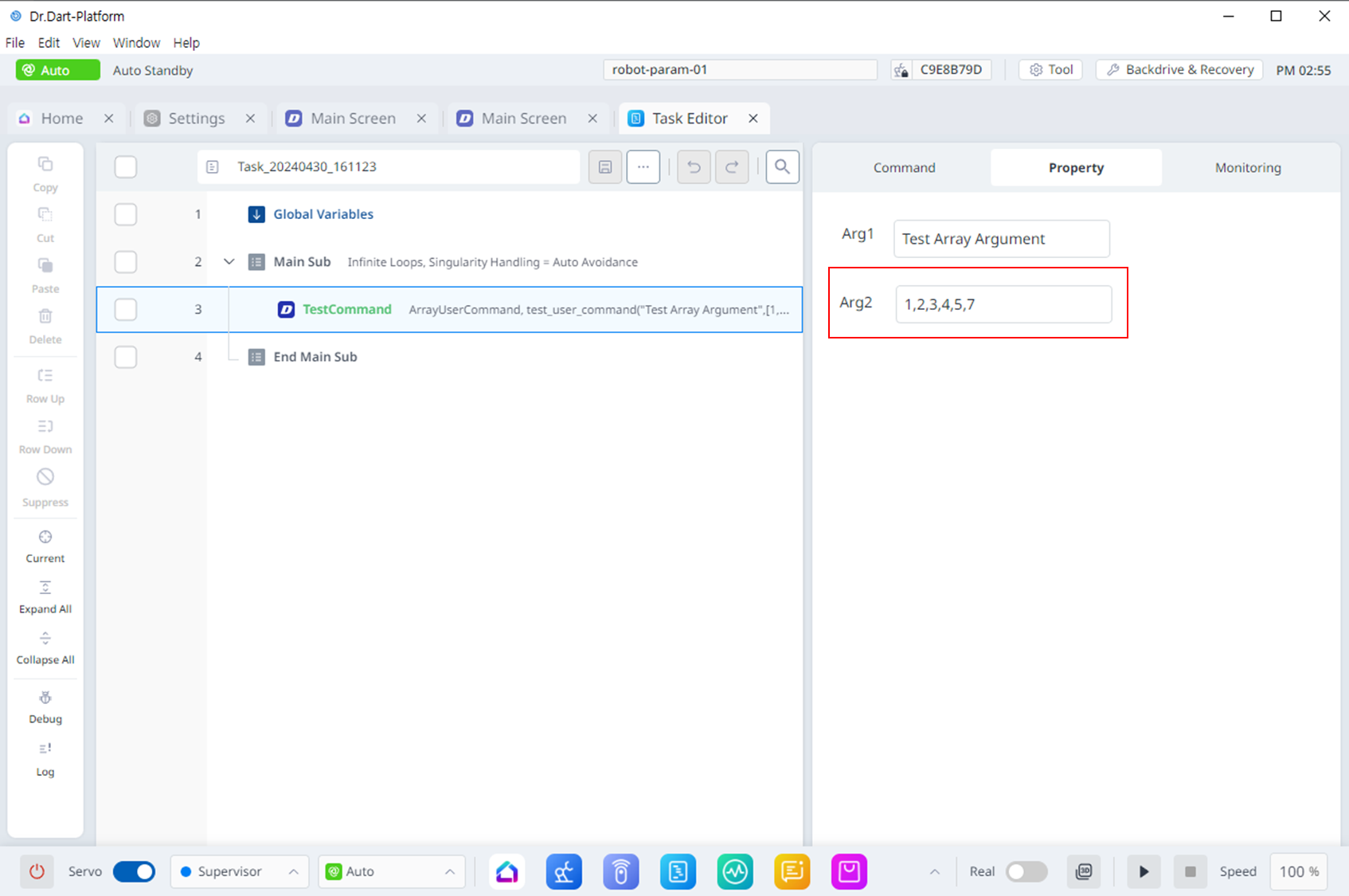
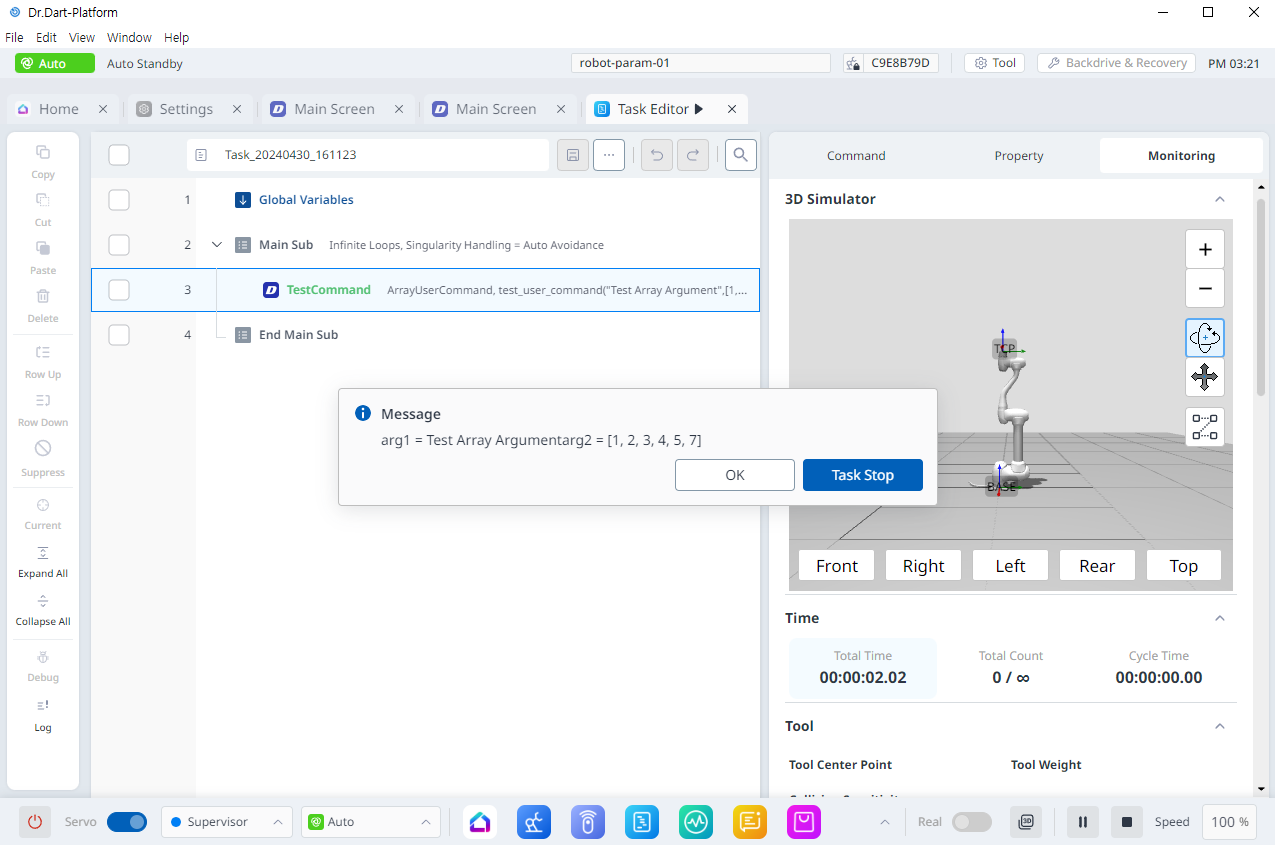