Table View
Overview
Table View shows all of the components that must be included in one table. You can re-design Table View to approach your goal.
Table View includes TableContainer, Table, TableHead, TableRow, TableCell, TableBody, TablePagination, Checkbox, TextField.
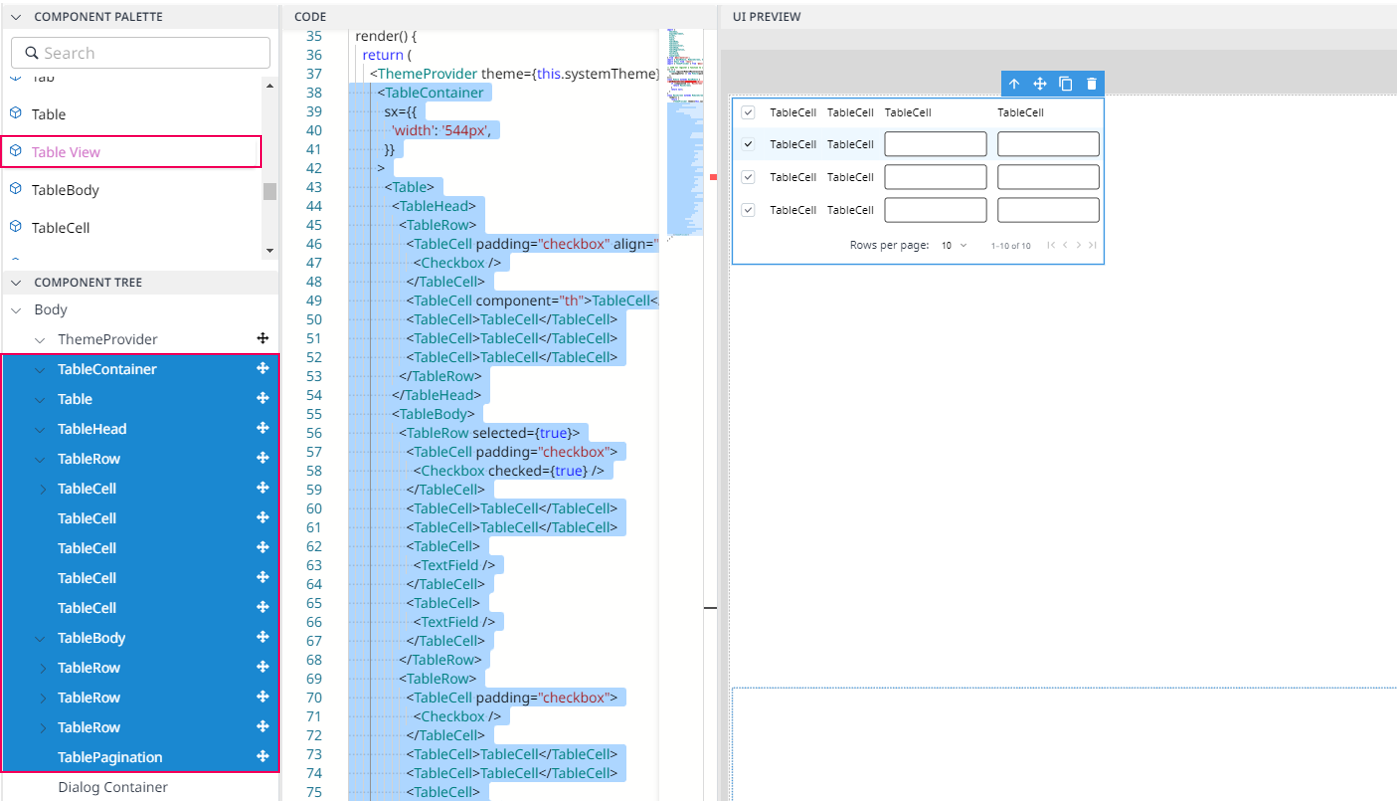
Import
CODE
import {
Checkbox,
Table,
TableBody,
TableCell,
TableContainer,
TableHead,
TablePagination,
TableRow,
TextField,
} from '@mui/material';
The above source will be imported automatically after dragging and dropping the Basic Component to the UI Preview Panel.
Code Sample:
CODE
<TableContainer
sx={{'width': '544px',}}>
<Table>
<TableHead>
<TableRow>
<TableCell padding="checkbox" align="center">
<Checkbox />
</TableCell>
<TableCell component="th">TableCell</TableCell>
<TableCell>TableCell</TableCell>
<TableCell>TableCell</TableCell>
<TableCell>TableCell</TableCell>
</TableRow>
</TableHead>
<TableBody>
<TableRow selected={true}>
<TableCell padding="checkbox">
<Checkbox checked={true} />
</TableCell>
<TableCell
sx={{'backgroundColor': 'yellow',}}>
Option 1
</TableCell>
<TableCell
sx={{'backgroundColor': 'orange',}}>
Option 2
</TableCell>
<TableCell>
<TextField />
</TableCell>
<TableCell>
<TextField />
</TableCell>
</TableRow>
<TableRow>
<TableCell padding="checkbox">
<Checkbox />
</TableCell>
<TableCell
sx={{'backgroundColor': 'blue',
'color': 'white',}}>
Option 3
</TableCell>
<TableCell>Option 4</TableCell>
<TableCell>
<TextField />
</TableCell>
<TableCell>
<TextField />
</TableCell>
</TableRow>
<TableRow>
<TableCell padding="checkbox">
<Checkbox />
</TableCell>
<TableCell>Option 5</TableCell>
<TableCell>Option 6</TableCell>
<TableCell>
<TextField />
</TableCell>
<TableCell>
<TextField />
</TableCell>
</TableRow>
</TableBody>
</Table>
<TablePagination
count={10}
rowsPerPage={10}
page={0}
onPageChange={() => {}}>
</TablePagination>
</TableContainer>